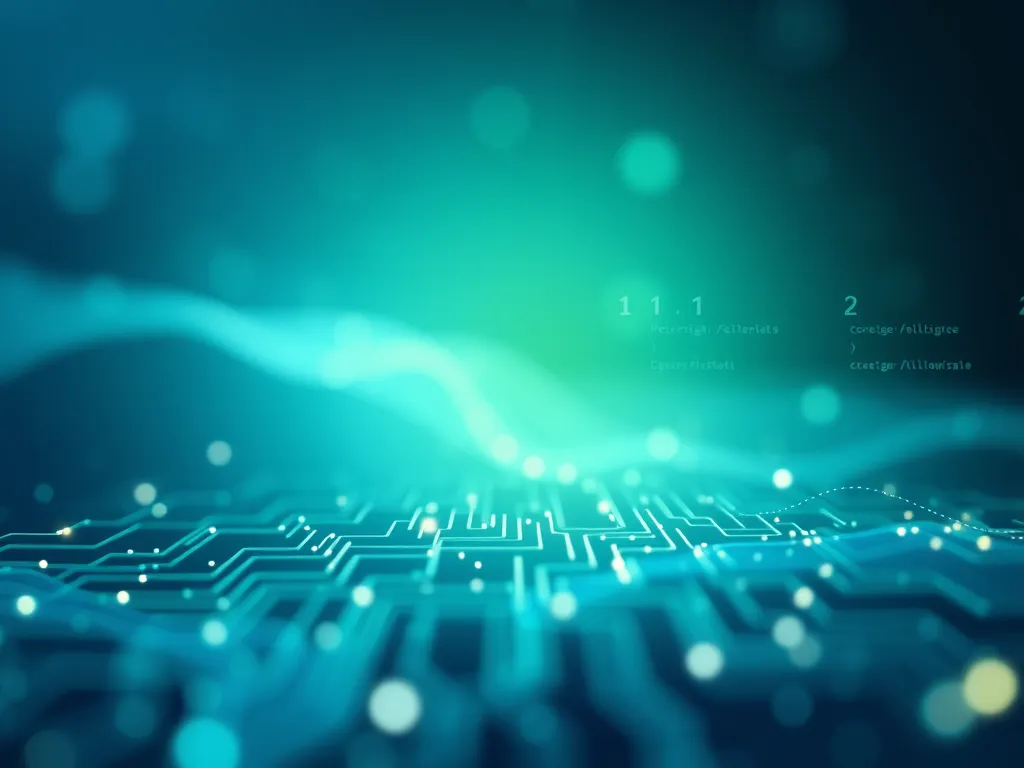
Background Task
Understanding Background Tasks in Software Development
Defining Background Tasks and Their Purpose
Background tasks are processes that run independently of the main application flow, allowing applications to perform operations without blocking user interactions. They are designed to manage operations that do not require immediate user feedback, such as data processing, file uploads, notifications, and periodic tasks. The relevance of background tasks in modern applications lies in their ability to enhance overall performance and responsiveness. By offloading resource-intensive operations to background threads, developers can create smoother user experiences while maintaining the integrity and functionality of the application's primary features.
The Significance of Background Tasks for User Experience
The importance of background tasks in software development cannot be overstated. They play a crucial role in improving user experience and overall efficiency of applications. By executing time-consuming tasks in the background, developers ensure that the user interface remains responsive, leading to higher user satisfaction. Additionally, background tasks contribute to efficient resource management by optimizing the usage of system resources, which enhances the performance of the entire system. Overall, the implementation of background tasks is essential for creating scalable and user-friendly applications.
Exploring Different Types of Background Tasks
Scheduled Tasks
Scheduled tasks are designed to run at predetermined times or intervals. These tasks are typically executed by a task scheduler, which can manage when and how often the tasks should run. Common use cases for scheduled tasks include performing database backups, sending out periodic email newsletters, and running batch jobs for data processing. In many systems, you can define schedules using cron expressions, allowing for flexibility in timing. For instance, a scheduled task might be set to run every night at midnight to update reports based on the previous day's data, ensuring users always have access to the latest information without manual intervention.
Asynchronous Tasks
Asynchronous tasks allow operations to run concurrently, freeing up the main thread from waiting for a function to complete. They are especially beneficial in environments where tasks can take a significant amount of time, as they enable a smoother user experience by keeping the application responsive. Asynchronous programming is achieved through approaches such as callbacks, promises, or async/await patterns. For example, in a web application, an asynchronous task might handle file uploads or external API calls without freezing the interface, thus improving performance and user satisfaction. The primary advantage of asynchronous tasks is their efficiency, enabling systems to maximize resource utilization and reduce overall latency.
Event-Driven Tasks
Event-driven tasks operate on a trigger-response model, executing actions in response to specific events. This type of task implementation is common in reactive programming where changes in state, user actions, or messages from other systems trigger the background processes. Event-driven architectures can optimize performance by responding dynamically to real-time changes, making them suitable for applications requiring immediate feedback, such as chat apps or interactive dashboards. A real-world example would be an e-commerce platform that triggers an order confirmation email immediately after a successful purchase, ensuring customers receive timely updates. Event-driven tasks enable systems to be more fluid, efficient, and aligned with user interactions or system states.
Background Task Type | Description | Use Cases | Key Benefits |
---|---|---|---|
Periodic Background Task | Executes a task at regular intervals | Data synchronization, updates | Efficient resource management, automation |
Long-Running Background Task | Processes that run over an extended period without user intervention | File uploads/downloads, batch processing | Improved user experience, offloads work from UI |
Background Task with Cancellation | Allows tasks to be canceled when no longer needed | User-initiated tasks | Reduces unnecessary processing, saves resources |
Asynchronous Background Task | Runs tasks in a non-blocking manner, enabling responsiveness | API calls, I/O operations | Enhances application performance |
Hosted Service Background Task | Leverages ASP.NET Core services for background processing | Scheduled jobs, message processing | Centralizes task management, utilizes built-in capabilities |
Implementing Background Tasks in Different Frameworks
Implementing Background Tasks in ASP.NET Core
- Setting Up the Project
-
Start by creating a new ASP.NET Core project using the command:
dotnet new webapp -n BackgroundTasksExample
-
Navigate into your project folder:
cd BackgroundTasksExample
-
Adding Background Service
- Create a new class called
MyBackgroundService.cs
in theServices
folder. - Implement the
IHostedService
interface and override theStartAsync
andStopAsync
methods:
csharp public class MyBackgroundService : BackgroundService { protected override async Task ExecuteAsync(CancellationToken stoppingToken) { while (!stoppingToken.IsCancellationRequested) { // Your background task logic here await Task.Delay(TimeSpan.FromSeconds(30), stoppingToken); } } }
- Register the Background Service
- Open
Startup.cs
and register your background service in theConfigureServices
method:
csharp
services.Add hostedService
- Running the Application
-
Run the application your console using:
dotnet run
Implementing Background Tasks in Android
- Creating a New Project
- Open Android Studio and create a new project. Choose the "Empty Activity" template.
-
Name your project and set the minimum API level.
-
Add Dependency for WorkManager
- Open
build.gradle
(Module) and add the WorkManager dependency:
groovy implementation "androidx.work:work-runtime-ktx:2.7.1"
- Create a Worker Class
- Create a new class
MyWorker
that extendsWorker
:
java public class MyWorker extends Worker { public MyWorker(@NonNull Context context, @NonNull WorkerParameters params) { super(context, params); }
@NonNull
@Override
public Result doWork() {
// Your background task logic here
return Result.success();
}
}
- Scheduling the Work
- In your
MainActivity
, schedule the background task usingWorkManager
:
java WorkRequest workRequest = new OneTimeWorkRequest.Builder(MyWorker.class).build(); WorkManager.getInstance(this).enqueue(workRequest);
- Running the Application
- Run your application on an emulator or device to test the background task functionality.
Effective Strategies for Managing Background Tasks
Monitoring Background Tasks and Effective Error Handling
Monitoring background tasks is essential to ensure they are running smoothly and efficiently. Implementing a robust logging system allows you to track the execution of tasks and capture any unexpected behavior. Utilize tools like Application Insights or custom logging frameworks to record metrics such as execution time, failure rates, and resource usage.
In addition to logging, setting up alerts for failures or performance degradation enables prompt action to rectify issues. Consider implementing retries with exponential backoff for transient errors, thus minimizing disruption. In scenarios where data integrity is critical, leverage transactional operations to ensure consistent states even when errors occur.
Another important aspect of error handling is creating clear error messages and categorizing them based on severity. This categorization aids in prioritizing which issues require immediate attention and helps in diagnosing problems quickly. By establishing a systematic approach to monitoring and handling errors, you can greatly enhance the reliability of background processes.
Tips for Optimizing Background Task Performance
Optimizing the performance of background tasks not only improves responsiveness but also conserves system resources. Start by analyzing the tasks to identify bottlenecks—focus on computationally expensive operations and consider offloading them to more efficient processes or using asynchronous programming techniques.
Make sure to leverage appropriate thread pools and adjust the concurrency model based on workload characteristics. Frequently, a task can be split into smaller sub-tasks that can run concurrently, thus reducing overall execution time. Also, configure the scaling of resources based on demand, allowing systems to handle peak loads dynamically without over-provisioning.
Minimizing I/O operations is another critical strategy. Opt for batch processing where feasible, which reduces the number of read/write cycles and improves throughput. Lastly, caching results of expensive operations can significantly lower resource usage and speed up repetitive tasks. By implementing these optimization strategies, you can enhance the efficiency and effectiveness of your background tasks.
Common Challenges and Solutions
Resource Management Challenges in Background Tasks
Background tasks are a crucial component of modern applications, yet they often face significant resource management issues. One common challenge is the increased consumption of CPU and memory resources, which can lead to application slowdowns and degraded user experience. This is primarily due to the asynchronous nature of background tasks, which may conflict with foreground processes.
To mitigate these issues, developers can implement resource throttling techniques. By limiting the number of concurrent background tasks, applications ensure that system resources are adequately allocated, preventing excessive resource consumption. Additionally, utilizing task prioritization allows developers to designate critical tasks and manage their execution order effectively, reducing the impact on overall performance.
Another common resource management issue involves handling task failures gracefully. Background tasks can fail for numerous reasons, including network issues or insufficient resources. Employing retry mechanisms with exponential back-off strategies can help ensure that transient errors do not lead to persistent failures. Furthermore, leveraging monitoring tools to track resource usage can provide insights into task performance, enabling developers to make data-driven adjustments.
Effective Debugging Techniques for Background Tasks
Debugging background tasks presents unique challenges, primarily due to their detachment from user interfaces. One effective technique is to implement extensive logging within the tasks. By logging the start and end of tasks, as well as any intermediate states or errors, developers gain visibility into task execution flow, making it easier to trace issues.
Another approach is to use specialized debugging tools that support background task execution. For instance, tools that allow for remote debugging can enable developers to attach directly to a running background process, providing real-time insights into the application's state. Setting breakpoints and inspecting variables in this environment can facilitate a deeper understanding of task behavior under various conditions.
Unit testing is also an essential practice in debugging background tasks. By writing comprehensive unit tests for task logic, developers can catch errors early in the development process. More specifically, using mock frameworks can simulate various environments and dependencies, allowing for thorough testing of task execution paths without the need for full application deployment.
Incorporating these techniques can significantly enhance the reliability and maintainability of background tasks, ultimately leading to better application performance and user satisfaction.
FAQs about Background Tasks
What are Some Common Examples of Background Tasks?
Background tasks are processes that run behind the scenes without requiring direct user interaction. Here are several examples: - Data Syncing: Applications often perform data synchronization with servers when there is a stable internet connection to keep local data updated. - File Uploads/Downloads: Certain apps allow users to upload or download files in the background, enabling continuous use of the app while these tasks are processed. - Notifications: Background tasks can be used to periodically fetch and send push notifications to the user, keeping them informed without active engagement. - Batch Processing: Large data sets can be processed in the background, freeing up resources and ensuring that the user interface remains responsive.
How Do Background Tasks Differ from Foreground Tasks?
Background tasks and foreground tasks are distinct in their execution and user interaction: - User Interaction: Foreground tasks require user input or attention, such as filling out forms or navigating through screens, while background tasks operate independently. - Resource Allocation: Foreground tasks often have priority access to CPU and memory, ensuring quick responses. In contrast, background tasks may be throttled to conserve resources and avoid degrading the foreground experience. - Use Cases: Background tasks are suitable for operations like fetching data, running updates, or syncing, while foreground tasks are used for interactive activities where user input is required.
Can Background Tasks Affect App Performance?
Yes, background tasks can significantly influence app performance and user experience: - Resource Contention: If not managed properly, background tasks can consume CPU and memory resources, which might slow down foreground tasks and lead to a laggy user experience. - Battery Consumption: On mobile devices, excessive or poorly optimized background tasks can drain battery life, affecting user satisfaction. - User Notifications: If background tasks are tied to sending frequent notifications, they can interrupt users, potentially leading to frustration if not balanced appropriately.