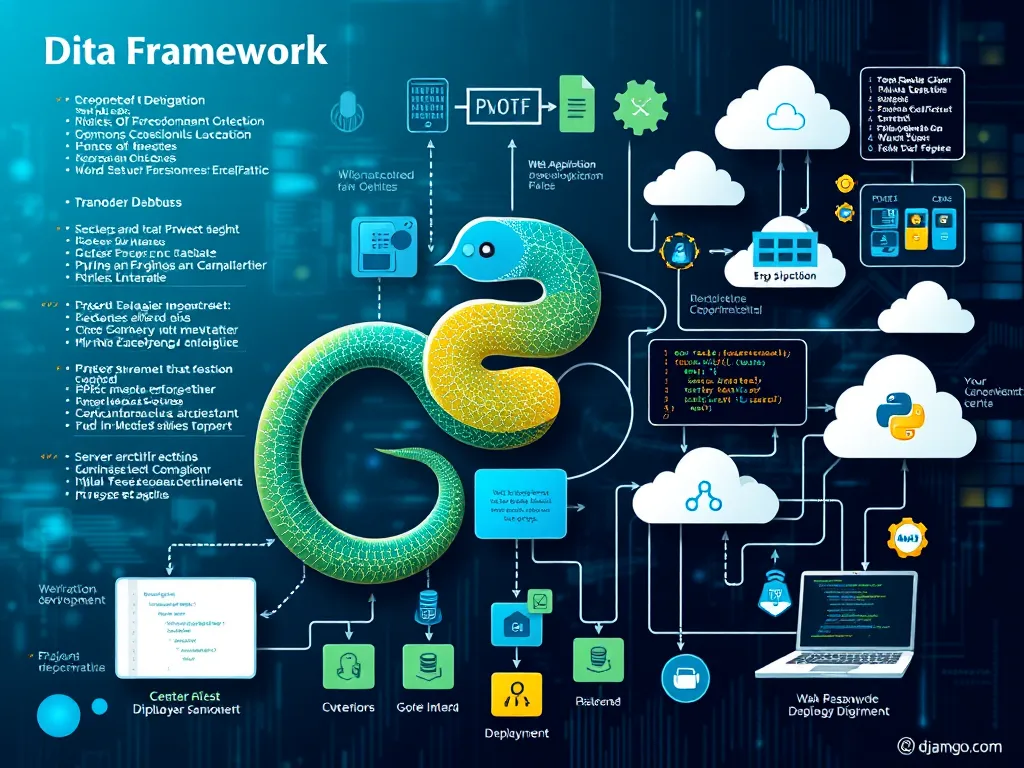
Getting Started with Django
Getting Started with Django can transform your web development journey, making it easier to build complex and maintainable applications. Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Whether you're a beginner or an experienced developer, this guide will provide you with the foundational steps to start using Django effectively.
To embark on your journey of Getting Started with Django, it’s essential to understand its core principles and the advantages it offers. Django follows the “batteries-included” philosophy, meaning it comes with many built-in features that streamline web development. This includes an admin panel, ORM (Object-Relational Mapping), authentication support, and much more, enabling you to focus on building your application rather than reinventing the wheel.
This guide will walk you through the initial setup, including how to install Django, create your first project, define models, set up views and templates, work with forms, and implement user authentication. By the end of this article, you will have a solid understanding of how to build a functional web application using Django.
The first step in Getting Started with Django is to familiarize yourself with its architecture and essential components. Django's architecture follows the Model-View-Template (MVT) pattern, which separates the data (Model), the logic (View), and the presentation layer (Template). This separation makes it easier to manage applications and promotes reusability and scalability.
In summary, Getting Started with Django offers an exciting path to mastering web development. With its powerful features and strong community support, it can significantly enhance productivity and streamline the development process. Now, let’s dive into the basics of Django and how you can set it up on your machine.
Django Basics
Django's architecture is designed around the Model-View-Template (MVT) pattern. In this structure, the Model represents the data, the View contains the business logic, and the Template is responsible for the presentation layer. This modular approach makes it easier to maintain and develop web applications as each component can be modified independently without affecting the others.
In today's digital age, choosing a service that is both Ridiculously fast. and reliable is essential for success.
To get started with Django, you first need to install it on your machine. Ensure you have Python installed (preferably version 3.6 and above). You can install Django using pip, the package manager for Python. Simply run the command: `pip install django` in your terminal or command prompt. This command retrieves the latest version of Django and installs it, making it ready for your use.
Once Django is installed, you can create your first Django project using the command: `django-admin startproject myproject`. This command creates a new directory named `myproject` containing the necessary files and directory structure to get you started. You can navigate into your project directory and run the server with `python manage.py runserver` to see your new Django project in action at `http://127.0.0.1:8000/`.
Django Models
In Django, models are Python classes that define the structure of your database. Each model class corresponds to a single table in the database. You can define fields and behaviors of the data you’re storing, and Django automatically generates the necessary SQL to create and manipulate database records based on these models.
Django’s Object-Relational Mapping (ORM) system provides a powerful way to interact with the database. You can use Python code to retrieve, insert, update, and delete records without writing raw SQL queries. For example, you can create a new entry in a model using `MyModel.objects.create(field1=value1, field2=value2)` which simplifies data handling significantly.
One important aspect of working with models in Django is database migrations. Migrations are changes that you apply to your database schema based on modifications to your model classes. You can create migrations with the command `python manage.py makemigrations` and apply them using `python manage.py migrate`. This ensures that your database stays in sync with your models.
Django Views and Templates
Views in Django are responsible for processing requests and returning responses. Each view function receives a web request and returns a web response. You can create views in Django by defining Python functions or classes that encapsulate the logic for your application. Views retrieve data from the model layer and pass it to the template layer to render the final output.
Templates in Django are HTML files that define how the output of your views will be presented to the user. By using Django’s templating language, you can include dynamic content, loop through data, and apply conditional logic directly in your HTML files. This makes it easy to create user-friendly web pages that display data from your application.
Django’s template syntax supports various filters and tags to enhance the rendering process. For instance, filters allow you to modify variables in the template, such as formatting dates or numbers. You can use the `{{ variable|filter }}` syntax to apply filters to your output. Understanding this syntax is crucial for creating dynamic web pages that interactively present information.
Django Forms
Forms are an essential part of web applications, allowing users to submit data to your server. Django makes creating forms easy by providing the `django.forms` module, which you can use to define form fields and layout. You can create a form in Django by creating a class that inherits from `forms.Form`, defining fields as class attributes, and customizing the layout as needed.
Validating form data in Django is straightforward. When you submit a form, you can use the `is_valid()` method to check if the data adheres to the rules defined for each field. For example, you can specify whether a field is required, must be a specific type (like an email), or should be within a certain range of values. This built-in validation helps maintain the integrity of your application's data.
Handling form submissions is another vital aspect of Django forms. You can create views to process form submissions, saving the data to the database or rendering the form again if validation fails. Django provides convenient methods to render forms in your views, ensuring users receive immediate feedback when interacting with your application.
Django Authentication
User authentication is crucial for most web applications. Django comes with a built-in authentication system that supports user registration, login, logout, and password management out of the box. To implement user authentication, you can use Django’s built-in views and forms, significantly reducing the time spent on security and user management.
Implementing permissions and groups in Django allows you to control user access to various parts of your application. You can create groups, assign permissions to those groups, and then assign users to them. This ensures that only authorized users can access sensitive areas of your application, enhancing security and usability.
Using Django’s built-in authentication system is straightforward. It includes everything from user management to password hashing and security checks. You can leverage the provided views for login and logout processes, and customize them as needed. Overall, Django’s authentication framework streamlines user management, allowing you to focus on building your application instead of dealing with complex security implementations.